How much do you need those Google Maps API features anyway?
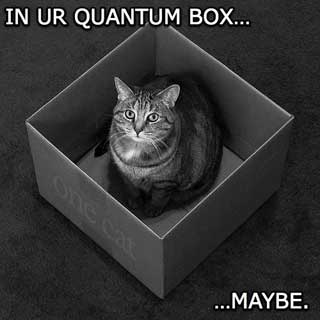
Like lots of people in our field, I have been contemplating moving off of the Google Maps API. I am in no way in danger of hitting the usage caps or violating the API’s TOS. I just have better data. With tools like TileMill/Mapnik I can make (I believe) better cartography. I can make a snappier app with Leaflet. I’d have more flexibility. And there would be no way a future Google Maps API TOS change could screw me.
But…there are some nice things about the API that are awfully hard/impossible to replace. Street view is a big one. Traffic is a big one. Google Earth integration (as hacky as it is with the v3 API) is a big one.
To gauge the level of protest I might encounter should I jump off the Google Maps API, I decided to do a little experiment. For the past week I have been capturing how often people use those features on GeoPortal. Now I can compare those numbers with Google Analytics to see how many people are taking advantage of those features and may show up at my door with torches and/or pitch forks.
First, how did I do that? Pubsub.
/* Some google maps related metrics */ function sendCounter(counter) { $.ajax({ type: "POST", url: "counter.php", data: { name: counter } }).done(function( msg ) { console.log( "Data Saved: " + msg ); }); } function watchAnything() { sendCounter("anything"); $.unsubscribe("/watch/anything", watchAnything); } function watchEarth() { sendCounter("earth"); $.publish("/watch/anything"); $.unsubscribe("/watch/earth", watchEarth); } function watchStreetview() { sendCounter("streetview"); $.publish("/watch/anything"); $.unsubscribe("/watch/streetview", watchStreetview); } function watchTraffic() { sendCounter("traffic"); $.publish("/watch/anything"); $.unsubscribe("/watch/traffic", watchTraffic); } $.subscribe("/watch/anything", watchAnything); $.subscribe("/watch/earth", watchEarth); $.subscribe("/watch/streetview", watchStreetview); $.subscribe("/watch/traffic", watchTraffic);
The first function there is what writes to the database. The rest of the functions are subscribed to a specific “/watch/*”. Each unsubscribes itself as soon as it fires once. So, in something like the streetview change position event, I’ll have:
$.publish("/watch/streetview");
Then the watchStreetview function fires. It writes to the database, publishes “/watch/anything”, and unsubscribes itself. The watchAnything function also writes to the database and unsubscribes itself. In this way each application load will only fire a particular usage once, and I’ll capture whether anything was used as well so I can roughly tell if people use more than 1 feature. This kind of dynamic coupling is what makes Pubsub so awesome.
The data gets written to a SQlite database. The PHP for that is painfully simple.
/** * Upload data to sqlite */ try { //open the database $db = new PDO('sqlite:.\counter.db'); //insert some data... $count = $db->exec("UPDATE counter SET " . $_REQUEST["name"] . " = " . $_REQUEST["name"] . " + 1"); // close the database connection $db = NULL; } catch(PDOException $e) { print 'Exception : '.$e->getMessage(); }
Yes, I know I’m totally SQL-injection vulnerable there. That’s one of the nice things about throw-away SQLite databases: I don’t care.
That code and making the SQLite database took all of an hour. Now on to the good stuff.
Over the course of a week, GeoPortal got 4,582 unique visitors and 7,168 page views. That’s enough people to do a reasonable calculation. The type of user on GeoPortal should also be a good cross-section of regular users and “power” users (whatever the hell that means). Because there is only 1 page in GeoPortal, a page view represents an app session and will translate 1:1 with the counts we accumulated in the experiment.
Here’s the final analysis:
Info | Count |
---|---|
App Sessions | 7,168 |
Used any of the features: Streetview, Traffic, Earth | 160 |
Used Earth | 68 |
Used Streetview | 73 |
Used Traffic | 35 |
Used more than 1 feature (estimate) | 16 |
% Users that will Come for my Head | 2.2-3.5% |
There were a few surprising results. I was surprised that Earth was so popular, almost as much as Streetview, particularly because it doesn’t show up for people that don’t have the Google Earth extension installed. I thought Streetview would be a lot more popular than it is. Traffic wasn’t too surprising.
But I found what I was expecting: those hard-to-replace Google Maps API features are not terribly popular. I can shift away from the Google Maps API and effect only a tiny percentage of my users. 3% of 4,582 is only 137 people. And if less than 500 people in a given week show up at my door with torches and/or pitch forks, it’s pretty clear what would happen.
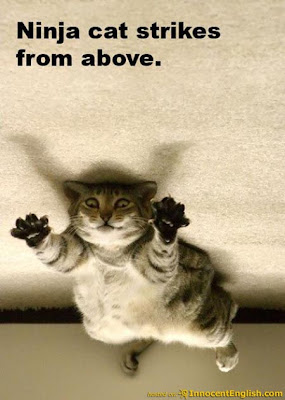