Google Earth API Integration via GeoWebCache
If you are local to the Mecklenburg County NC area and use our GeoPortal a lot, you might have noticed something new lately.
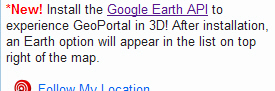
If you have the Google Earth API installed, you get this in your list of overlays:
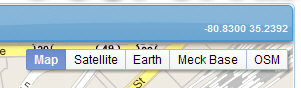
Should you be brave enough to click the Earth button, behold!
One of the best things that happens at conferences are the conversations you have with other attendees. On the shuttle ride to the airport I happened to sit next to somebody that works at Google on the Fusion Tables project and had the opportunity to hit him with a few questions. One of those questions was when the Google Earth API was going to catch up to and be integrated with Google Maps API v3. I didn’t get a direct answer on that (my guess is they’re planning a WebGL replacement of the plugin down the road), but he did point me to a great script that helps integrate the two, including transferring overlays from one to the other. Perfect!
Except for one thing. It transfers every Google Maps overlay but the one I use most often - ImageMapType. GAAAA!
Fortunately, GeoWebCache can serve regionated KML tiles, and the Google Earth API supports that. A little fancy footwork and I can get our layers showing up on Google Earth in the browser.
I ended up monkeying about with the original script a lot, including tossing some things I didn’t need, so I’d recommend starting with the original as a base. Here’s basically what I did:
I made a new function called addMeckLayers and added it to the refresh function.
1 | GoogleEarth.prototype.refresh_ = function() { |
I also declared a global folder var, which I set in the initializeEarth function.
1 | GoogleEarth.prototype.initializeEarth_ = function() { |
Here’s the function. A lot of this is very specific to my app, so just use it as a baseline. I’m also rocking some jQuery in there, which won’t help if you aren’t doing so also. First I dump the folder contents. Then I loop through the layer control back on the main page, and if the layer’s active and it has a kmlnetworkpath property set in my config, I add the layer to the folder. Then I add the parcel lines (they’re always on) and add the folder the map. Bob’s your uncle.
1 | /** |
There’s more going on here and there in the app. I attach the opacity slider and layer control to Earth if it’s active. I also get the elevation/lat/lng on mouse move via:
1 | // Mouse move event listener for coordinate display |
You can get my whole unsquished script here, but again, I’d start with the original and work from there. I’ll add it to the GeoPortal open source template when I can pry a couple of screaming projects off of my desk.
One thing to note about GeoWebCache’s KML. It doesn’t use the proxy settings from GeoServer inside the KML, it uses the the requesting host URL. That means if you’re using and Apache proxy pointing to GeoWebCache on localhost:8080, your KML will have localhost:8080 embedded. That will lead to disappointment. Your Apache config needs this to fix it:
[crayon lang=”default”]
ProxyPreserveHost On